Experience AWS Lambda function development from a .NET developer's point of view
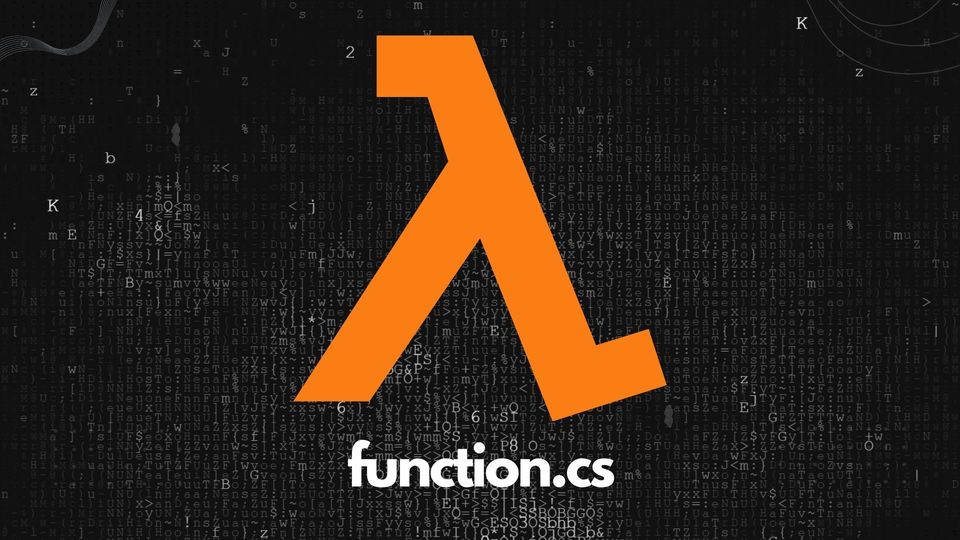
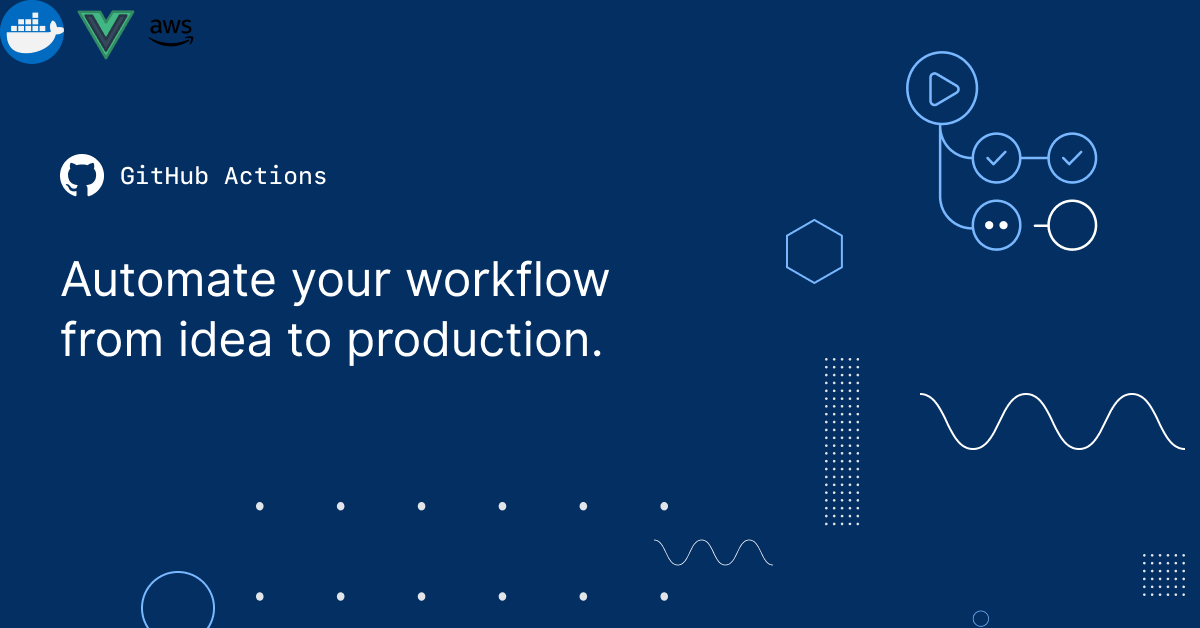
AWS recently added .NET 6 runtime support to lambda function and it encourage me to do some practice to see how well AWS support developer, just like me! This supposed to be a short article, we will quickly review the experiences when developing, debugging & publishing lambda function using .NET 6 & Visual Studio 2022.
The IDE and project creation.
Let's start with our beloved IDE (Integrated Development Environment), in this post I used Visual Studio Enterprise 2022, but other versions are supposed to provide the same experience.
First, VS 2022 IDE doesn't natively support AWS development, we need to install "AWS Toolkit for Visual Studio 2022" from the Visual Studio marketplace before starting development (https://marketplace.visualstudio.com/items?itemName=AmazonWebServices.AWSToolkitforVisualStudio2022). It quite easy to add the toolkit via a few clicks, and new AWS project template will appear after VS IDE restarted.
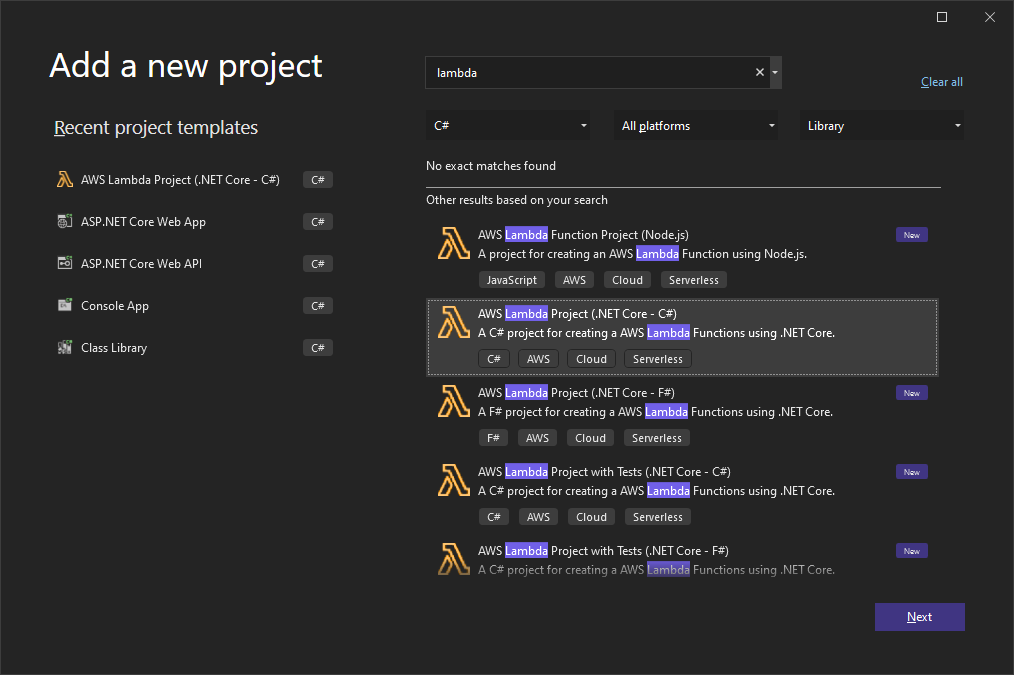
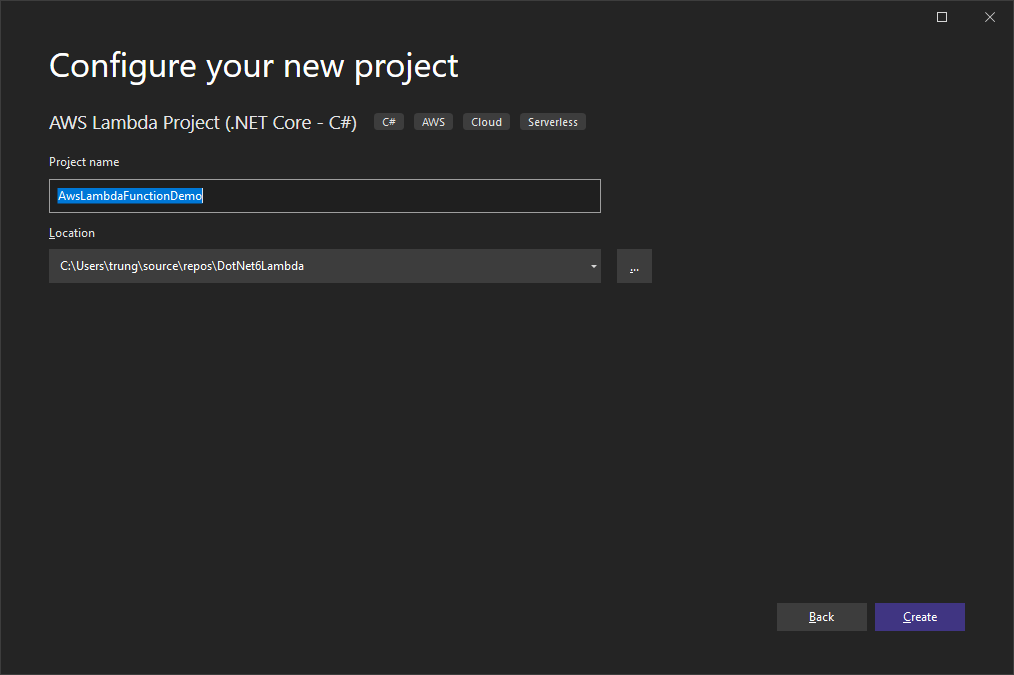
Let's create a new project using a pre-defined project template (AWS Lambda Project (.NET Core - C#)), then click Next. Named it "AwsLambdaFunctionDemo" or use whatever name you like then press Create.
In this step, we can select some blueprints from AWS, which covered popular scenarios. I decided to try the Simple S3 Function (react to any events from S3 buckets), and click Finish to complete the project creation process.
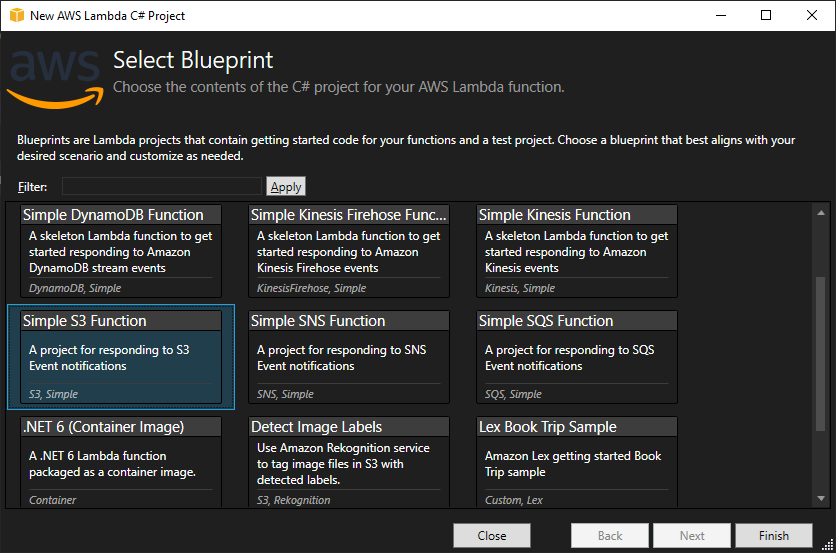
The new project was created with a very simple structure:
- "Function.cs" file which defines the lambda function behavior
- aws-lambda-tools-defaults.json: lambda function configurations information
I can also notice some built-in packages like S3 SDK or Lambda Core. As it is simple, it just took a few milliseconds to build (what is good!).
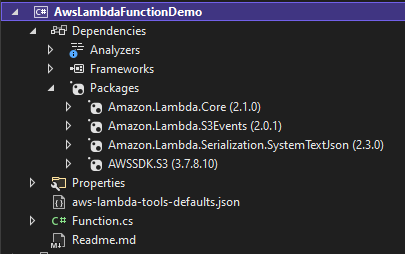
The function handler code is simple as well, and we noticed the support from AWS for logging (an important part of our application), the Logger is well-configured and can be accessed via context.Logger.
public async Task<string?> FunctionHandler(S3Event evnt, ILambdaContext context)
{
var s3Event = evnt.Records?[0].S3;
if(s3Event == null)
{
return null;
}
try
{
var response = await this.S3Client.GetObjectMetadataAsync(s3Event.Bucket.Name, s3Event.Object.Key);
return response.Headers.ContentType;
}
catch(Exception e)
{
context.Logger.LogInformation($"Error getting object {s3Event.Object.Key} from bucket {s3Event.Bucket.Name}. Make sure they exist and your bucket is in the same region as this function.");
context.Logger.LogInformation(e.Message);
context.Logger.LogInformation(e.StackTrace);
throw;
}
}
Test & debugging locally
After successfully creating a new lambda function project, my next question is how can we test and debug the function locally? And I think AWS make a good job here, they created something called the "Lambda mock test tool", which allows you to mimic the same testing experiences as AWS Console.

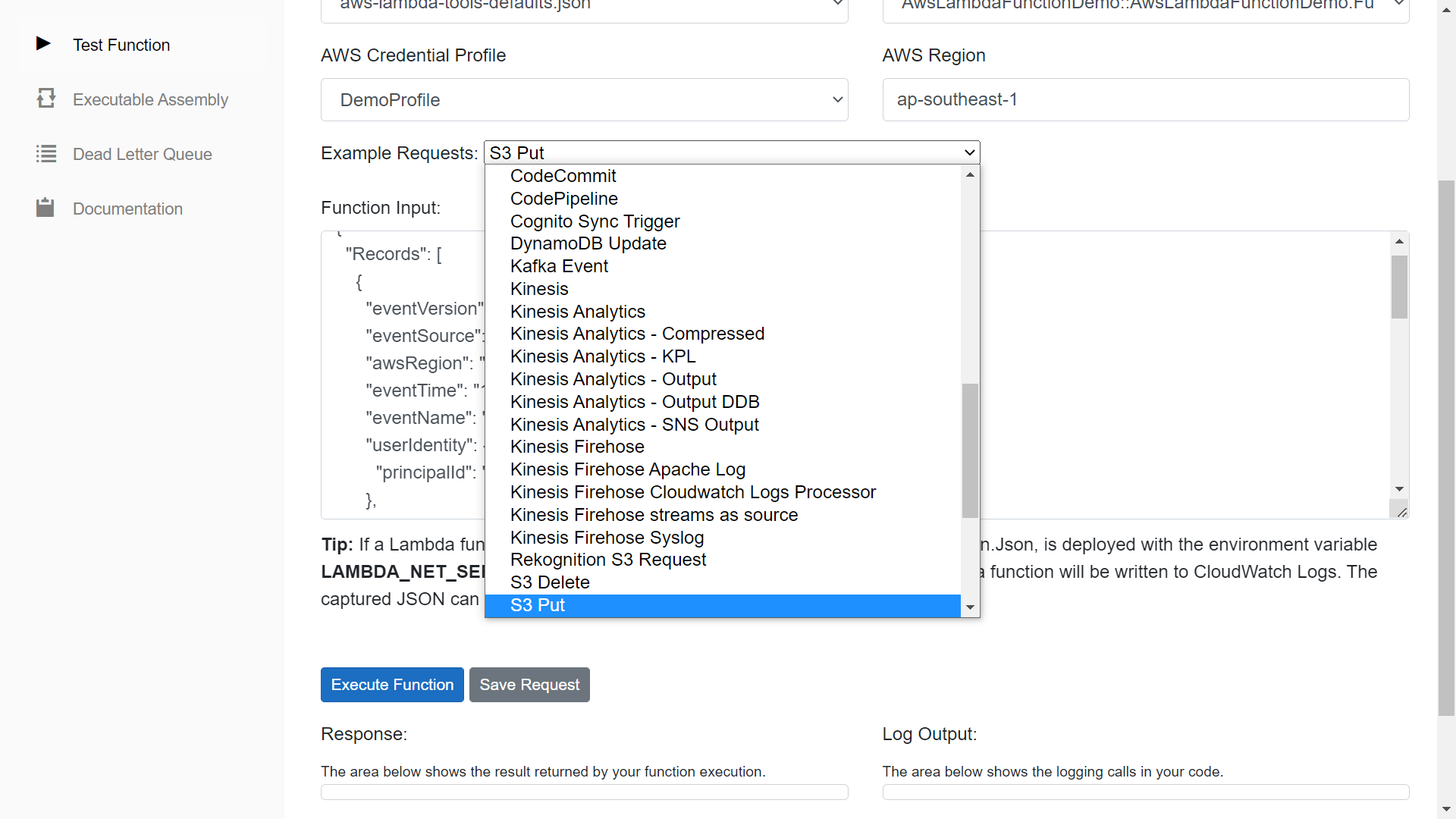
After starting the lambda function locally, you can select the predefined example requests or put any requests in the Function input field and click "Execute Function" to trigger the lambda. What a convenient and sufficient way!
You can also set the breakpoint at the function handler like any other .NET application.
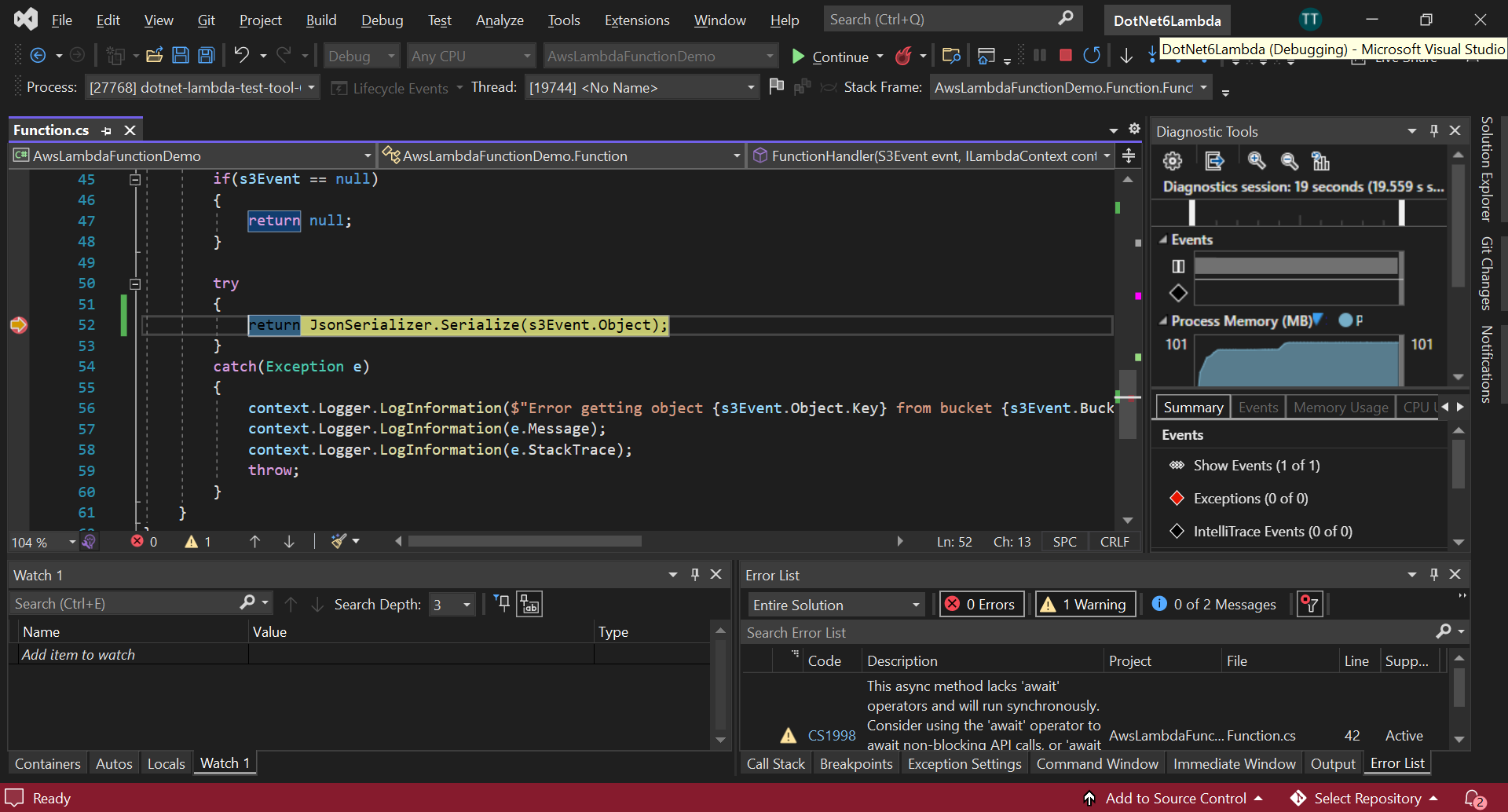

Publish to AWS
The final part of this review will be the publishing part. Before doing that, we need to configure a profile, that will be used by the visual studio to publish our application. Go to View/AWS Explorer and select Add AWS credential profile.
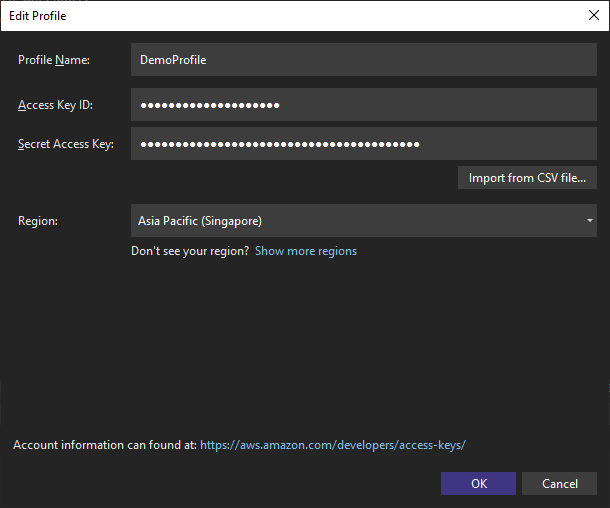
Then we can right-click on the lambda project name and select Publish to AWS Lambda to start publishing the function from VS 2022 IDE.

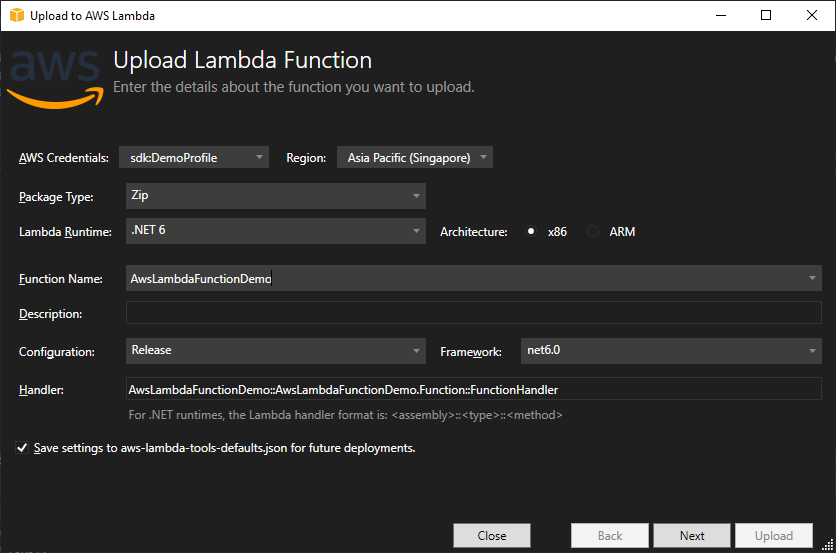
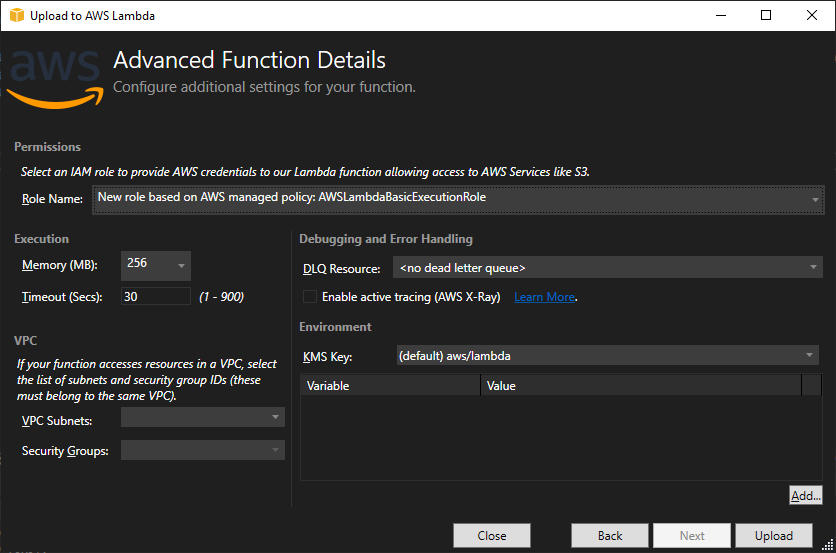
After filling in all required information, click Upload to complete the process. After a few seconds, check out AWS Console and noticed a new lambda function was created. The next part is out of the article's scope so I will stop there.

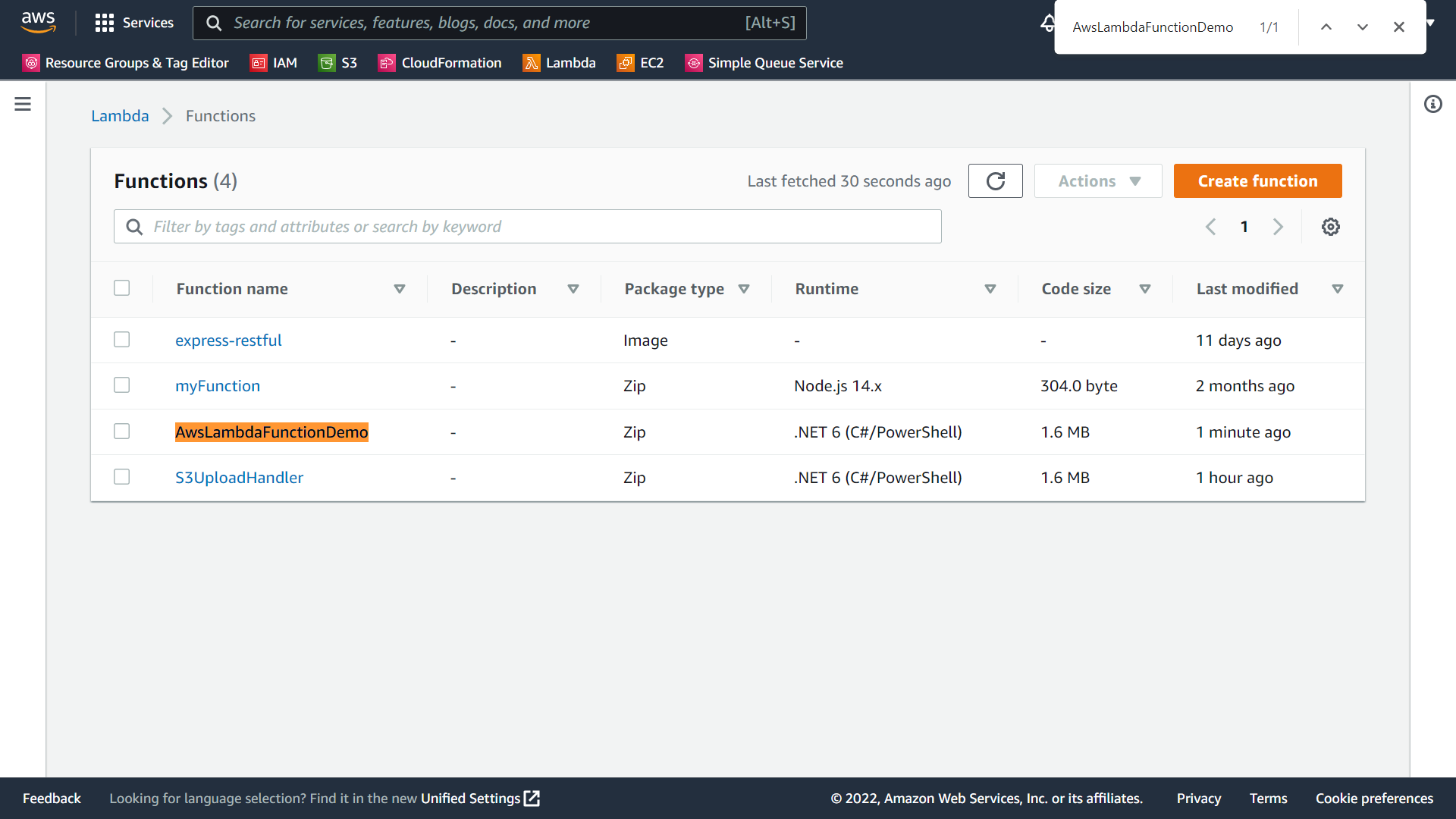
Conclusions
What I like:
- The setup process is simple and easy to follow, even for the newbie!
- The testing and debugging experiences are stunning (even better than the traditional application :D)
- Easy to publish the function to AWS!
What I don't like:
- Not much, maybe I need to spend more time and find a more complex demo. I may come back and update this post later if I find some!
References: https://aws.amazon.com/blogs/compute/introducing-the-net-6-runtime-for-aws-lambda/?nc1=h_ls